To store the entire list we use a 2d array of strings in C language. The array of characters is called a string. “Hi”, “Hello”, and e.t.c are the examples of String. Similarly, the array of Strings is nothing but a two-dimensional (2D) array of characters. To declare an array of Strings in C, we must use the char data type. Sentence 1: 'Finally you can both initialize and size your array, as in mySensVals.' - sentence 2: 'Note that when declaring an array of type char, one more element than your initialization is required, to hold the required null character.' Both sentences are true, but only the first refer to mySensVals. I am facing a problem with allocation of two dimensional char array. The problem statement requires me to get the number of rows and columns of the array from a file. I have saved the rows in 'rows' and columns in 'columns', both of type int. Here is how i initialized the array. Char.array; array = new char. String and Character Array. String is a sequence of characters that is treated as a single data item and terminated by null character ' 0'.Remember that C language does not support strings as a data type. It only works for a one-dimensional array if the initial value is zero - because the elements that are not explicitly initialised are initialised to zero. To do what you want (set all characters in the 2D array to 'V') you need to use a loop that assigns the elements individually. Or, possibly, use memset.
Contents- C++ Initialize Array
C++ Initialize Array
To initialize a C++ Array, assign the list of elements separated by comma and enclosed in flower braces, to the array variable. Initialization can be done during declaration itself or later in a separate statement.
In this tutorial, we will go through some examples of how to initialize arrays of different datatypes.
C++ Array Initialization during Declaration
Method 1 Cara membuka file bin.
2d Array In C
You can assign the list of elements to the array variable during declaration. If you do not specify the size of array, the number of elements you are initializing with becomes the size of array.
In the following example, we have not specified any size in the square brackets.
But, as we have assigned a list of five elements to the array, the size of the array becomes 5
.
Method 2
You can also specify the size of array, and assign a list of elements to the array. The number of elements in the list could be less than the size specified. Then, the compiler creates array with specified size, and assigns the elements one by one from the starting of array.
C# Initialize 2d Array
We have specified the array size to be 10
. But we have given only seven elements in the initializing list. So, the first seven elements of the array get initialized with the given list of elements, and the rest of array elements are initialized to zero.
Method 3
You can also declare an array with a specific size and initialize elements later individually.
inta[10]; a[1] =3; a[3] =2; |
C++ Multidimensional Char Array Initialization
C++ Initialize Array of Integers
In this example, we initialize two arrays of integers: one array with size not specified during declaration and the second with size specified and fewer elements initialized.
C++ Program
#include <iostream> //initialize array with no size specified //initialize array with size specified, but with fewer elements for (inti=0; i<sizeof(arr1)/sizeof(*arr1); i++) { } for (inti=0; i<sizeof(arr2)/sizeof(*arr2); i++) { } |
Output
73872 |
C++ Initialize Array of Strings
Aiptek hyperpen 8000u pro. In this example, we initialize two arrays of strings: one array with size not specified during declaration and the second with size specified and fewer elements initialized.
C++ Program
#include <iostream> //initialize array with no size specified stringarr1[] = {'hello' , 'hi', 'good' , 'morning'}; //initialize array with size specified, but with fewer elements stringarr2[10] = {'hello' , 'hi', 'good' , 'morning', 'thank', 'you', 'user'}; //print arrays for (inti=0; i<sizeof(arr1)/sizeof(*arr1); i++) { } for (inti=0; i<sizeof(arr2)/sizeof(*arr2); i++) { } |
Output
hello hi good morning |
C++ Initialize Array of Chars
In this example, we initialize two arrays of chars: one array with size not specified during declaration and the second with size specified and fewer elements initialized.
C++ Program
#include <iostream> //initialize array with no size specified //initialize array with size specified, but with fewer elements chararr2[10] = {'t', 'u', 't', 'o', 'r', 'i', 'a', 'l'}; //print arrays for (inti=0; i<sizeof(arr1)/sizeof(*arr1); i++) { } for (inti=0; i<sizeof(arr2)/sizeof(*arr2); i++) { } |
Output
aeiou |
C++ Initialize Array of Floating Point Numbers
In this example, we initialize two arrays of floating point numbers: one array with size not specified during declaration and the second with size specified and fewer elements initialized.
C++ Program
#include <iostream> //initialize array with no size specified floatarr1[] = {7.98652, 5.7443, 8.88888, 4.1255557, 2.22222}; //initialize array with size specified, but with fewer elements floatarr2[10] = {5.882, 5.578, 1.448, 92.232, .74965, .6669, 888.884}; //print arrays for (inti=0; i<sizeof(arr1)/sizeof(*arr1); i++) { } for (inti=0; i<sizeof(arr2)/sizeof(*arr2); i++) { } |
Output Hindi movie pukar 2000.
7.986525.74438.888884.125562.22222 |
C++ Initialize Array of Long
In this example, we initialize two arrays of long numbers: one array with size not specified during declaration and the second with size specified and fewer elements initialized.
C++ Program
#include <iostream> //initialize array with no size specified longarr1[] = {798652, 57443, 888888, 41255557, 222222}; //initialize array with size specified, but with fewer elements longarr2[10] = {5882, 5578, 1448, 92232, 74965, 6669, 888884}; //print arrays for (inti=0; i<sizeof(arr1)/sizeof(*arr1); i++) { } for (inti=0; i<sizeof(arr2)/sizeof(*arr2); i++) { } |
Output

7986525744388888841255557222222 |
C++ Initialize Array of Booleans
In this example, we initialize two arrays of boolean values: one array with size not specified during declaration and the second with size specified and fewer elements initialized.
C++ Program
#include <iostream> //initialize array with no size specified //initialize array with size specified, but with fewer elements boolarr2[10] = {true, false, false, false, false, true, true}; //print arrays for (inti=0; i<sizeof(arr1)/sizeof(*arr1); i++) { } for (inti=0; i<sizeof(arr2)/sizeof(*arr2); i++) { } |
Output
11010 |
Conclusion
C++ New Char Array
In this C++ Tutorial, we learned how to initialize an array in C++ in different ways, and for different datatypes, with example C++ programs.
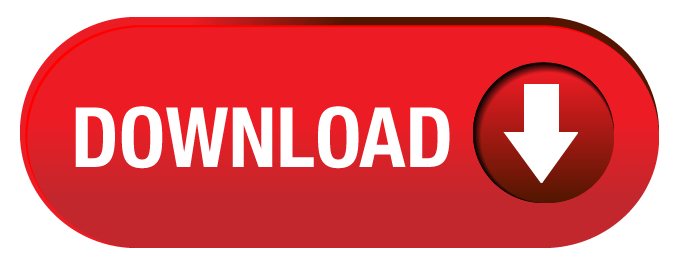